Introduction
The following sections give a simple overview how to make a simple TIM API integration without any special configurations. Therefore default values are used as often as possible to keep it simple. If special features are required, visit the section Integration in Detail for a more sophisticated overview.
Library usage
As the TIM SDK offers the TIM API in multiple languages you can chose the one that is suitable for your platform.
To use the TIM API Javascript there are two deliverables required, the "timapi.wasm" WebAssembly module and the "timapi.js". They can be used in two ways:
npm install websocket
Another speciality of the TIM API JavaScript is that the API offers a onTimApiReady() callback function which must be implemented by the integrator. This function is called as soon as the WebAssembly part of the TIM API has finished loading and the TIM API is ready to be used by the user. Therefore, the integrator can start using the TIM API after the onTimApiReady() call:
/*
* This function "onTimApiReady" will be called as soon as the TimApi is ready
* to be used.
*/
onTimApiReady = function() {
// Start using the TIM API, e.g. by initializing
// the required objects, e.g. TerminalSettings / Terminal
}
Minimal Settings
To start right away only a minimal set of settings has to be given:
- Terminal IP: The IP of the terminal that shall be used afterwards. Used if direct connection is used: ConnectionMode = OnFixIp
AutoCommit
: Defines if terminal performs a Commit automatically or if it must be done from the ECR. (Default: AutoCommit = true)
This minimal configuration can be done directly in the code after creating aTerminalSettings
instance.
This block shows the setup with the fixed ip connection mode:
// Create new TerminalSettings instance
let settings = new timapi.TerminalSettings();
// ----------------------------CONNECTION----------------------------
// IP address of the terminal.
settings.connectionIPString = "192.168.1.10";
// Port to be used for connection.
// Port list:
// 80 - Standard port for ws:// connection
// 8080 - If ports below 1024 are privileged and cannot be used.
settings.connectionIPPort = 80;
// ----------------------------COMMIT----------------------------
// If the ECR shall be responsible to perform a commit the following parameter has to be set.
// Otherwise the terminal performs a commit automatically.
settings.autoCommit = false;
// ----------------------------CREATE TERMINAL INSTANCE----------------------------
// Create a terminal instance using the adjusted terminalSettings object
var terminal = new timapi.Terminal(settings);
After the Terminal instance has been created it can be used to perform transactions.
Simple Transaction
The flow on the right shows a simple transaction flow and which steps are done by which participant. Note that for a simple use the integrator only needs to follow 4 simple steps, steps 1 to 4. Steps 1-4 can be performed multiple times (loop) if wished. The rest, steps A to G, will be done either by the TIM API itself or by the terminal if required.
The steps the integrator has to perform are the following:
- 1. Start transaction: The integrator has to call the
transactionAsync
function including the required parameters which are shown in the code example below. - 2. Process transaction completed: After processing the transaction the TIM API will return a
transactionResponse
in the correspondingtransactionCompleted
callback method. - 3. Send commit (if AutoCommit deactivated): After receiving the feedback in the transactionCompleted callback the integrator has to call the
commitAsync
function to complete the transaction process. This step must only be done if AutoCommit has been set to "false" in the terminal settings. - 4. Process commit completed (if AutoCommit deactivated): Also the commit function is finished from the TIM API by calling the
commitCompleted
callback function. After getting the commit completed callback call from the TIM API, another requests, e.g. another transaction, can be called.
The following steps are performed by the TIM API or the terminal if they are required:
- A. : The TIM API automatically connects to the terminal and makes the required Login to set the
specified connection parameters. If successful a shift will be opened on the terminal by calling Activate from the TIM API automatically.
These steps are only performed if they are required which means the terminal was not yet in the corresponding state and not yet ready to accept a transaction:
- If the terminal is already connected, no connect is required.
- If the terminal is already in LoggedIn state, no login is required.
- If the terminal is already in Activated state (open shift), no activate is required.
- B. : After the TIM API performed all steps needed to bring the terminal into the required state, the transaction request is sent to the terminal.
- C. : The terminal performs the transaction (e.g. cardholder verification, transaction authorization etc.)
- D. : The terminal returns the outcome of the transaction to the TIM API.
- E. : The TIM API sends the commit request to the terminal (will be done automatically, if AutoCommit is activated).
- F. : The terminal processes the commit request.
- G. : The terminal sends the commit response to the TIM API.
In code this may look as follows:
exampleEcr = {};
// Create a customized listener-class extending the DefaultTerminalListener
class mylistener extends timapi.DefaultTerminalListener {
terminalStatusChanged(terminal) {
super.terminalStatusChanged(event, data);
// Do Stuff
}
transactionCompleted(event, data){
super.transactionCompleted(event, data);
//Do Stuff
}
}
function initTimApi(){
// Define all required settings
let settings = new timapi.TerminalSettings()
// Minimal settings
settings.connectionIPString = "192.168.1.10";
settings.connectionIPPort = 80;
settings.autoCommit = false;
// The integrator id is provided by SIX Payment Services / Worldline
// to identify the integrator for support purposes.
settings.integratorId = "0e6b1705-ab96-455b-9ba3-a77dd919d7a5";
// Create terminal object with
exampleEcr.terminal = new timapi.Terminal(settings);
exampleEcr.terminal.setPosId("ECR-01");
exampleEcr.terminal.setUserId(1);
exampleEcr.terminal.addListener(new myListener());
}
//A standard purchase transaction can be implemented as follows
function purchase(amount, currency) {
try {
// Prepare a timapi amount object for the current transaction
let myAmount = new timapi.Amount(amount, currency);
// Perform the purchase transaction
terminal.transactionAsync(timapi.constants.TransactionType.purchase, myAmount);
} catch( err ) {
console.Log("Error:", err);
}
}
//To finalize a transaction and if AutoCommit = false,
//the commit function must be used. An implementation could
//look as follows.
function commit() {
try {
// Perform the commit
terminal.commitAsync();
} catch( err ) {
console.Log("Error:", err);
}
}
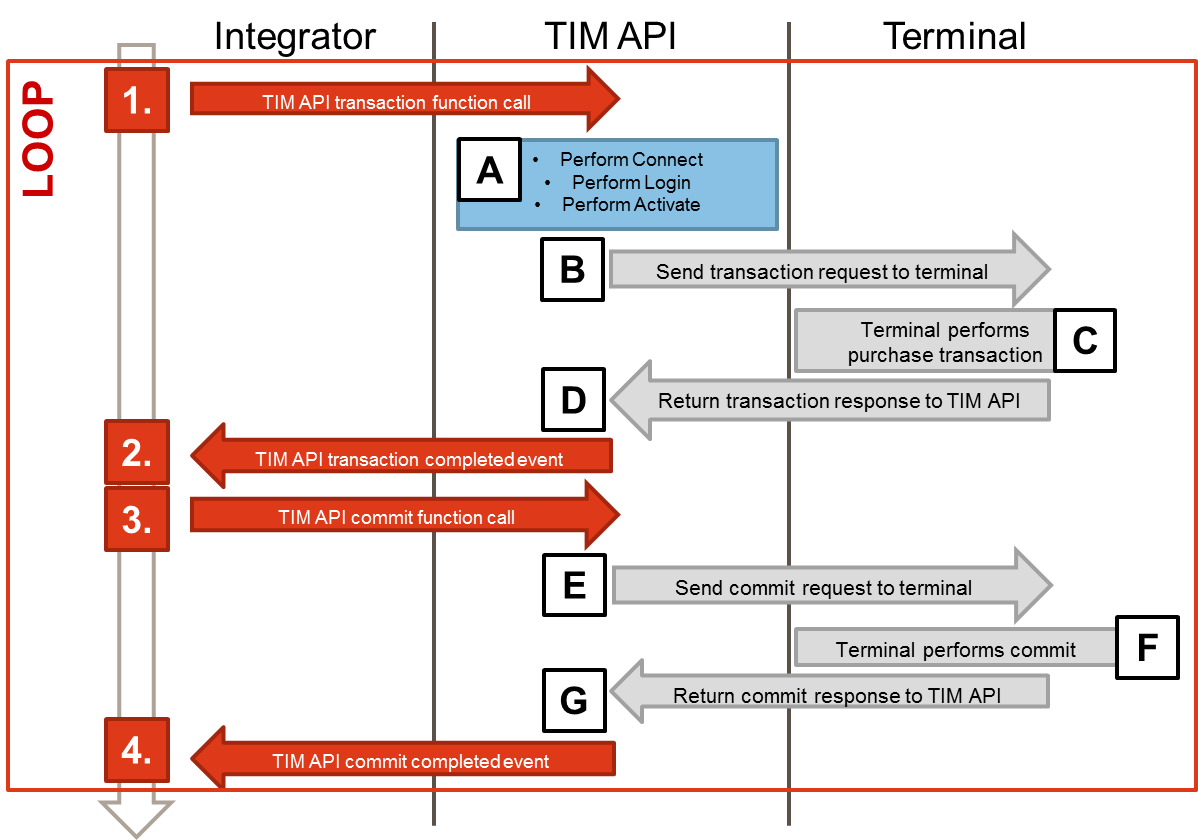
Simple Response Handling
The following section explains how to easily retrieve response data from the TransactionResponse
which is available after a transaction as described above.
The first step is to implement your own terminal listener class wherein you overwrite the functions you want to use. If you are mainly interested in the transaction response you can use the following code:
/** Asynchronous listener handling terminal events. */
/* Define your own TerminalListener by overwrite all required
* methods from the DefaultTerminalListener class
* which you want to use.
*/
class MyTerminalListener extends timapi.DefaultTerminalListener {
// Overwrite all wanted methods of the DefaultTerminalListener herein
}
/* Then create an instance of your "MyTerminalListener" and add it to
* your terminal instance.
*/
var myListener = new MyTerminalListener();
terminal.addListener(myListener);
Check transaction outcome
To check if a transaction has been performed successfully you have to check the ResultCode
which is part of the object in thetransactionCompleted
callback.
Further error information can be retrieved as described in the API documentation in the section.
Print data
The TransactionResponse
has a member PrintData
(accessed via printData
) which contains merchant and cardholder receipts.
The PrintData
returned in the
TransactionResponse
contains a merchant and
a cardholder receipt which can be retrieved in the transactionCompleted
callback using the printData
function. The PrintData
object
then contains a list of Receipt
objects which can be retrieved individually.
Card data
To retrieve card specific information the CardData
object is also available in
the TransactionResponse
. This object contains several card information that
was available to the terminal during the transaction process.
Further transaction information
Further information regarding the transaction can be found in the TransactionInformation
object.
The following code shows how the information mentioned above can be retrieved in the
transactionCompleted
event:
/* These completed functions have to be implemented in your "MyTerminalListener"
* class to overwrite the default implementation.
* You decide which functions you want to use.
*/
class MyTerminalListener extends timapi.DefaultTerminalListener {
transactionCompleted(event, data){
super.transactionCompleted(event, data);
/*--- Check transaction outcome ---------------------------------------*/
// Check if transaction has been successful
if (event.exception === undefined)
{
console.log("Transaction was performed successfully!");
/*--- Print data ------------------------------------------------------*/
// Further information can be retrieved as the transaction has be successful
// Get print data which contains a list of receipts
if (data !== undefined && data.printData !== undefined){
var myPrintData = data.printData;
// Go through all available receipts and print them accordingly
if(myPrintData.receipts !== undefined){
var myReceipts = myPrintData.receipts;
// If you want to differ bewteen merchant and cardholder receipt
for (var i = 0; i < myReceipts.length; i++) {
// Get merchant receipt
if(myReceipts[i].recipient == timapi.constants.Recipient.merchant){
console.log("Merchant receipt: ", myReceipts[i].value);
}
// Get cardholder receipt
if(myReceipts[i].recipient == timapi.constants.Recipient.cardholder){
console.log("Cardholder receipt: ", myReceipts[i].value);
}
}
}
/*--- Card data -------------------------------------------------------*/
// Get CardData which contains all available card information
if (data.cardData !== undefined){
var myCardData = data.cardData;
// Further card information can be retrieved out of the CardData object
// e.g. AID or BrandName etc...
var aid = myCardData.aid;
var brandName = myCardData.brandName;
}
/*--- Further transaction information ---------------------------------*/
// Further transaction information is available in the TransactionInformation object
if (data.transactionInformation !== undefined){
var myTrxInfo = data.transactionInformation;
// This object contains e.g. acquirer id or transaction reference and more information..
var acqId = myTrxInfo.acqId;
var acqTransRef = myTrxInfo.acqTransRef; // If available
var trmTransRef = myTrxInfo.trmTransRef;
}
/*---------------------------------------------------------------------*/
}
// If the transaction has not been successful the result code can
// be retrieved as follows to determine what went wrong
else
{
console.log("Transaction was NOT performed successfully:",
event.exception.resultCode);
}
}
}